How to Create Empty Function in Javascript
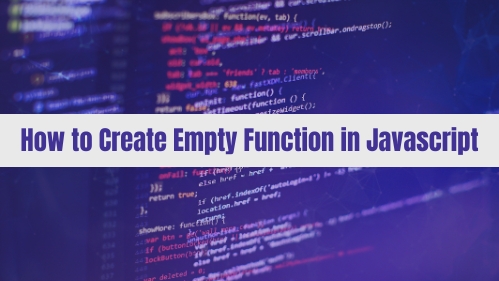
In JavaScript, functions play a crucial role in organizing and executing code. Sometimes, you may need to create a function without any specific code inside it initially. This is known as an empty function, which serves as a placeholder that you can later customize and fill with the desired functionality.
In this article, we will guide you through the process of creating an empty function in JavaScript, providing you with a foundation for building and expanding your codebase.
Learn how to create an empty function in JavaScript. This beginner-friendly guide provides step-by-step instructions for defining a function without any code block, allowing you to customize and add functionality as needed.
Step-by-Step Guide: Creating an Empty Function in JavaScript
Follow these steps to create an empty function in JavaScript:
Step 1: Declare the Function
To create an empty function, start by declaring it using the function
keyword, followed by the function name. For example, you can use the following syntax:
function myFunction() {
// Function body
}
Step 2: Customize the Function Name
Replace myFunction
with a name that describes the purpose of your function. Make sure to choose a meaningful and descriptive name that reflects its intended functionality.
Step 3: Add Optional Parameters
If your function requires parameters, you can include them within the parentheses after the function name. Parameters are variables that hold the values passed to the function when it is called. For an empty function, you can omit the parameters or include them based on your requirements. Here’s an example:
function myFunction(param1, param2) {
// Function body
}
Step 4: Leave the Function Body Empty
To create an empty function, simply leave the function body empty. This means there are no statements or code blocks within the curly braces {}
. An empty function acts as a placeholder that you can fill with code later. Here’s an example:
function myFunction() {
// Function body is empty
}
Step 5: Save and Use the Empty Function
After creating the empty function, you can save your JavaScript file and use the function within your codebase. The empty function can be called like any other function, allowing you to add code and functionality to it whenever required.
By following the step-by-step guide in this article, you can declare an empty function, customize its name, and optionally include parameters. Remember to leave the function body empty initially, allowing you to add statements and code blocks as your code evolves.