Convert SRT to Text with Regex and JavaScript
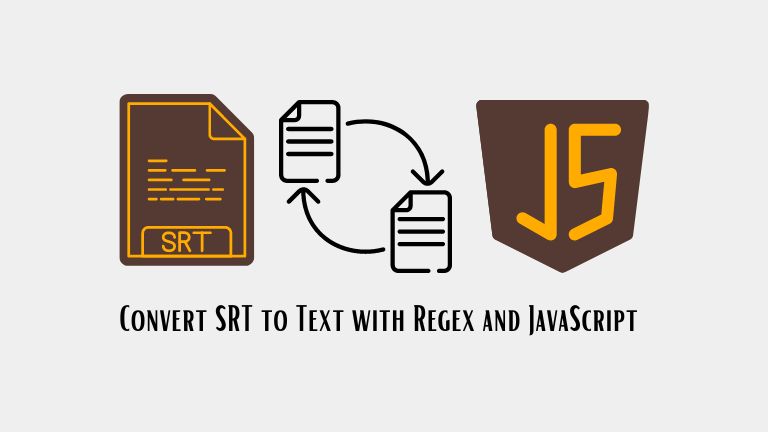
Subtitle files come in different formats, one of which is .srt, the most popular format for subtitles. If you are working with subtitles, you might need to extract the text from an .srt file. This article will guide you through the process of converting an .srt file into plain text using JavaScript and Regular Expressions (Regex).
Step 1: Understanding the SRT File Format
An .srt file is a plain text file that contains subtitles along with timing information. The format is simple and consists of several blocks of text, each of which represents a subtitle. Each block starts with a line containing a sequential number, followed by the start and end time of the subtitle in the format of “hh:mm:ss,ms”, and finally the subtitle text.
Here is an example of an .srt file:
1
00:00:20,000 --> 00:00:24,400
Welcome to this tutorial!
2
00:00:26,700 --> 00:00:29,100
Today we will learn about converting SRT to text.
3
00:00:30,500 --> 00:00:33,900
Let's get started!
Step 2: Reading the SRT File
To convert an .srt file into plain text, we need to first read its contents. We will use JavaScript’s File API to read the contents of the file. Here is the code to read the contents of an .srt file:
const input = document.getElementById("input");
const output = document.getElementById("output");
input.addEventListener("change", function () {
const reader = new FileReader();
reader.onload = function () {
const srt = reader.result;
convertSRTtoText(srt);
};
reader.readAsText(input.files[0]);
});
function convertSRTtoText(srt) {
// code to convert the SRT to text
}
Step 3: Using Regular Expressions (Regex)
to Extract the Text Now that we have the contents of the .srt file, we can use Regular Expressions (Regex) to extract the text from it. We will use the .split() method to split the contents of the .srt file into an array of blocks, and then use the .match() method to extract the text from each block.
Here is the code to extract the text from the .srt file:
function convertSRTtoText(srt) {
const blocks = srt.split("\n\n");
let text = "";
for (const block of blocks) {
const match = block.match(/(?<=\d\n)(.*)(?=\n)/);
if (match) {
text += match[0].replace(/[\d:\n\r]+/g, " ") + "\n";
}
}
output.value = text;
}
In this code, the Regular Expression /(?<=\d\n)(.*)(?=\n)/
is used to extract the subtitle text from each block. The (?<=\d\n)
and (?=\n)
parts of the Regular Expression are lookbehind and
lookahead assertions, respectively. They match a string without including it in the result. The .*
part of the Regular Expression matches any character (except for a newline) zero or more times, effectively capturing the subtitle text.
Step 4: Cleaning Up the Text Once we have extracted the subtitle text, we need to clean it up to remove any unnecessary information. In this case, we want to remove the start and end times of the subtitles, as well as any newline characters. We can use the .replace() method to do this.
Here is the code to clean up the text:
text += match[0].replace(/[\d:\n\r]+/g, " ") + "\n";
In this code, the Regular Expression /[\d:\n\r]+/g
matches one or more digits, colons, newline characters, or carriage return characters. The g
flag specifies that the match should be global, meaning it will replace all occurrences of the matched pattern, not just the first one. The replace method then replaces the matched pattern with a single space character.
Step 5: Displaying the Text
Once the .srt file has been converted to plain text, we can display it on the screen. We will use the .value property of the output element to set the text.
Here is the code to display the text:
output.value = text;
Conclusion
In this article, we have seen how to convert an .srt file into plain text using JavaScript and Regular Expressions (Regex) and guide to convert srt to text regex javascript. The process involves reading the contents of the .srt file, extracting the subtitle text using Regex, cleaning up the text to remove any unnecessary information, and finally, displaying the text on the screen. With this knowledge, you can now easily convert .srt files into plain text for further processing or analysis.